Last Friday, I added dark mode to W3Things, and announced that I'd be writing a tutorial on how you can also easily create and add dark mode for your website using just a few lines of code.
There are many ways of achieving that, however I'll focus on one of the simplest and easiest ways of doing so, sharing the same method and piece of code as I added to this site.
Let's get started.
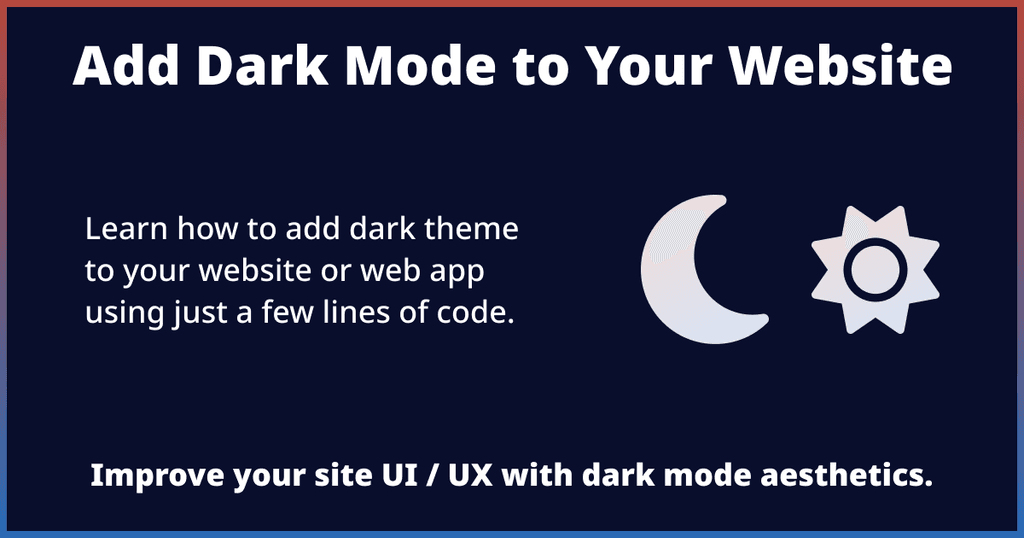
Our Requirements
To set our expectations and requirements clear for the following tutorial, we need to achieve the following with our dark theme.
- Add a theme switcher or theme toggle that allows the website users to switch the theme between light or dark as they prefer.
- Remember the theme preference (light or dark), so when a user revisits the site, the appropriate theme is automatically applied.
- Ensure that the entire code (HTML markup, CSS styles, JavaScript functionality) of the theme switcher is accessible and semantic.
- Keep the code as optimized and minimal as possible.
What We'll be Using
Like I said above, there are various ways in which you can create a dark mode for your website.
In this beginner's guide, we'll use the following things to add a dark and light mode to your website.
- TypeScript (or JavaScript): For switching or toggling between light and dark mode and remembering the user's theme choice.
- SCSS and CSS variables: For styling our theme switcher and creating the color schemes for both the light theme and dark theme.
- HTML: For adding light or dark mode toggle markup or controls.
Creating Dark Mode for Website
I'm assuming you already have an existing website or app where you want to implement dark mode.
If not, then you may create a new simple website or web page to follow along.
Step 1: Adding the HTML Markup for Toggle Switch
Copy and paste the following HTML code where you want to display the toggle buttons.
<div class="theme-switcher">
<label class="light" title="Light Theme">
<input name="theme" type="radio" value="light" title="Light Theme">Light
</label>
<label class="dark" title="Dark Theme">
<input name="theme" type="radio" value="dark" title="Dark Theme">Dark
</label>
</div>
Where you add the theme switcher depends on your website design, but it's always a good idea to add it in a prominent location where users can easily spot and access it.
I'd personally advise placing it on your site header or footer. This way, users can quickly and easily toggle the theme as needed.
Alternatively, you may also add the toggle in your site or app settings page like the way GitHub does it.
Step 2: Adding the SCSS (CSS Styles) for Light and Dark Color Schemes or Themes
Next, add the following theme switcher styles and color themes to one of your CSS stylesheets.
.theme-switcher {
border-radius: 8px;
background-color: #4d4d4d;
display: flex;
gap: 4px;
padding: 4px;
label {
display: flex;
gap: 4px;
padding: 8px;
align-items: center;
cursor: pointer;
border-radius: 8px;
color: #fff;
transition: all 150ms ease-in-out;
&:hover,
&:focus,
&:active {
background-color: #212121;
}
}
input[type='radio'] {
appearance: none;
position: absolute;
}
}
[data-theme='light'] {
.light {
background-color: #212121;
}
}
:root,
[data-theme='light'] {
--body-bg: #fff;
--text: #333;
--heading: #000;
}
[data-theme='dark'] {
.dark {
background-color: #212121;
}
--body-bg: #212121;
--text: #fff;
--heading: #fff;
}
Here, I've only added the styles for theme switcher and basic CSS variables as a starting point.
Of course, you'll need to add and adjust the colors and variable names depending on your website layout and design.
Step 3: Adding the TypeScript (JavaScript) Code for Toggling between Light and Dark Mode
Next, let's add the TypeScript code (or JS code if you're using JavaScript) to add the functionality for our inputs or buttons to toggle dark mode on your website.
export function themeSwitcher (): void {
const userTheme = localStorage.getItem('theme')
const switchers: NodeListOf<HTMLInputElement> = document.querySelectorAll('[name="theme"]')
switchers.forEach((switcher) => {
const switcherValue = switcher.value
if (switcherValue === userTheme) {
switcher.checked = true
}
switcher.addEventListener('change', () => {
document.documentElement.dataset.theme = switcherValue
localStorage.setItem('theme', switcherValue)
})
})
}
Understanding the TypeScript Code
In our TS code, we're exporting a simple function which will be called or invoked in our main TS bundle file. You may directly invoke the function in the same file if needed.
In the function, we have 2 variables - userTheme
and switchers
.
The userTheme
variable stores the value of the theme
key if it's available in the local storage property. We're doing this to check if the user has already chosen a theme on our site in a previous visit. Read more about this in step 4 below.
The switchers
variable stores all the occurrences of the elements that have the value of theme
in their name
attribute. These are our input radio buttons we added above in the HTML markup section.
Next, we're looping through our radio inputs using forEach
method.
In the loop block, we're using the switcherValue
variable to grab the value of the input (in this case, either light
or dark
).
In the following conditional block, we're simply marking the relevant radio input as selected which has the same theme value as our user's selected theme using the theme
key-value pair from the local storage API.
Then, we attach a change
event listener to our radio inputs so when the user changes the theme using the input toggles, we update the data-theme
attribute on our HTML element to the specified theme value.
In addition to updating the data attribute, we also update the value of our local storage theme
item accordingly (to light
or dark
).
Step 4: Adding the JavaScript Code for Remembering the Color Theme in the Browser
For a good user experience, we need to remember the user's theme choice even if they restart or close their browser.
We'll use one of the modern Web Storage APIs, the local storage or localStorage
web API.
This API allows us to store site data persistently, even when the browser is closed. Read more about the localStorage API on MDN.
Go ahead and add the following line of JavaScript code within your site's <head>
tag.
<script>(() => { const a = localStorage.getItem("theme"); a && (document.documentElement.dataset.theme = a) })();</script>
You might wonder why we're adding it to <head>
when we could also add it to our TS file or function.
The reason for doing so is to prevent the flash of unstyled content.
Understanding the JavaScript Code
Here, we have a single line of minified code that contains an Immediately Invoked Function Expression (IIFE).
In this code, we're initializing a variable called a
to access and store the value of the theme
item from the localStorage
property.
In the following line statement, we're checking if the variable a
is truthy. If it is truthy (has any value for the theme
key), we assign its value to the data-theme
attribute on our web document's HTML element.
Step 5: Testing the Dark Theme
Once you've completed all the previous steps, go ahead and check your website or app to see if everything works correctly.
Use the radio input to switch between the themes and see if all the colors are being applied appropriately.
You may need to adjust some Cascading Style Sheets variables for both themes if you find some elements looking odd or not adapting properly.
For a live test, you can always check this website, W3Things, with dark mode enabled to see how it looks and works.
Wrapping Up
That wraps up our beginner's guide for adding dark mode to your website.
If you have any questions or need help with a particular step, feel free to mention me on X (formerly Twitter) or get in touch via email at [email protected].
Be sure to subscribe to the newsletter to directly receive web development tutorials like this and more content in your inbox.
Frequently Asked Questions about Adding Dark Mode
How do I enable dark mode on my website?
Follow the above tutorial steps to enable dark mode on your website.
Should I make my website dark mode?
About a decade ago, having a dark mode for websites might seem like an optional feature or an afterthought.
But, in today's digital age of websites and web apps, having a dark mode or dark theme should be one of the fundamental features.
So yes, I personally believe your website should have the dark mode option.
How do I optimize my website for dark mode?
To ensure your website is optimized for dark mode, make sure that you first complete the light color scheme palette.
Once you've finalized everything for the light mode, then start making a dark mode palette, such as using a dark background and white text for the overall site look and feel.
Make sure that you change the colors as needed and avoid using too high contrast colors (such as pure black background color) to prevent strain on the eyes.
A good example is to look at some of the sites that have the dark theme like MDN or GitHub and see how they've defined their color palette.
If you still need help optimizing your website for dark mode, feel free to get in touch with me.