In this beginner's tutorial, we'll learn how to create a responsive two column and multi-column layout using the modern CSS properties, Flexbox and Grid.
While there are many ways to create such page layouts, these modern properties allow you to create multiple-column layouts in HTML without using JavaScript or any CSS frameworks like Bootstrap or Tailwind.
Furthermore, they are commonly used and serve as a handy tool for web developers in designing responsive web designs and complex website layout structures without writing dozens of lines of CSS codes.
Let's get started.
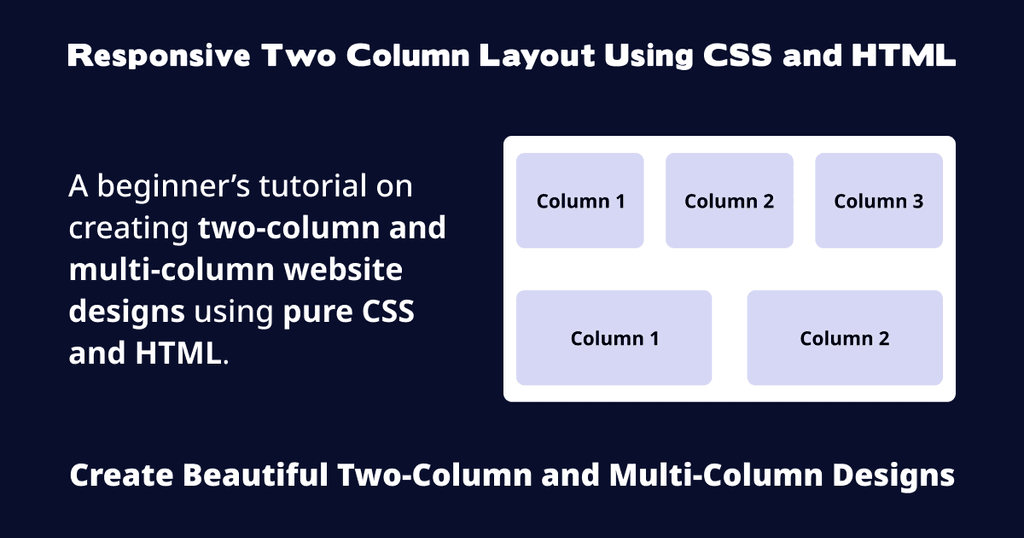
Two Column Layout with HTML and CSS Grid Layout
First, let's see how to achieve our desired outcome using the Grid method.
Creating a two-column design with it is straightforward and relatively simpler than Flex.
Step 1 - The HTML Markup (Using CSS Grid)
Open your preferred source code editor like VS Code or Atom.
Copy and paste the following HTML markup into a new file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Two Column Layout HTML</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="./index.css">
</head>
<body>
<main>
<section class="container">
<div class="column">This is column 1.</div>
<div class="column">This is column 2.</div>
</section>
</main>
</body>
</html>
Save the file as index.html
.
We'll use the same markup throughout this tutorial.
Understanding the Markup
Most of the markup is the bare minimum for rendering our page and should be self-explanatory. The lines we'll focus on are the following.
Line 14 is our parent container or wrapper for the Grid.
We've given it a class of container
to which we'll apply styles in the next step using Cascading Style Sheets (CSS).
Line 15 is our individual column with the class of column
.
Step 2 - The CSS Style Rules (Using CSS Grid)
As with the HTML markup above, we'll create a base CSS file that we'll reuse throughout this tutorial, albeit with some changes along the way.
body {
margin: 0;
padding: 1rem;
}
.container {
display: grid;
grid-template-columns: 1fr 1fr;
gap: 1rem;
}
.column {
padding: 1rem;
border-radius: 8px;
background-color: hsl(240, 62%, 90%);
}
@media (max-width: 1024px) {
.container {
grid-template-columns: 1fr;
}
}
Save the file as index.css
in the same directory as index.html
.
Understanding the Style Rules
Line 7 sets the section element (container
class) to display as the Grid layout.
Line 8 sets the number of columns to 2.
Both columns take up equal space with the help of the 1fr
value (more info on this is mentioned below).
Read more about the grid-template-columns syntax on MDN.
If you prefer, you can also use other CSS units, such as pixels or percentages.
Line 9 sets the gap between both columns to be 1rem
(equal to 16px
).
Here, we'll use CSS Gap for spacing, but you can also use margin or padding to create space between the columns.
Line 20 sets the grid wrapper to display 1 column on small screen sizes (in our case, equal to or below 1024px
).
The rest of the rules tidy up our columns and the page a bit, which should be self-explanatory.
Changing the Width of the Columns
In CSS Grid, fr
means a fraction of the leftover space in the wrapper.
So, if you prefer column 1 to be larger than column 2, use the following values.
grid-template-columns: 2fr 1fr;
You can read more about this unit in this MDN article.
Step 3 - Running the Website (Using CSS Grid)
Open the website (index.html
in our case) in your web browser, and you should see two columns laid out side-by-side with a purple background color on each.

If you resize your browser (equal to or below the 1024px
breakpoint) or view the website on a mobile device, you should see the columns stacked on each other.

2 Column Layout with CSS Flexbox and HTML
Creating a two-column layout with Flexbox requires a bit more work than Grid.
You have to apply some style rules to the child elements as well.
Step 1 - The HTML Markup (Using CSS Flexbox)
The markup we'll use for Flex is the same as the above.
Step 2 - The CSS Style Rules (Using CSS Flexbox)
Copy and paste the following CSS into a new file.
body {
margin: 0;
padding: 1rem;
}
.container {
display: flex;
flex-wrap: wrap;
gap: 1rem;
}
.column {
padding: 1rem;
border-radius: 8px;
background-color: hsl(240, 62%, 90%);
flex: 1;
}
@media (max-width: 1024px) {
.column {
width: 100%;
flex: auto;
}
}
Understanding the Style Rules
Most rules are similar, so we'll focus on the Flex-related changes here.
Line 7 sets the section tag (container
class) as the Flexible box layout.
Line 8 wraps the columns on small screens (equal to or below 1024px
in our case). This is done to prevent horizontal content overflow.
Line 16 allows the columns to grow or shrink within the Flex container depending on the available space with the help of the flex shorthand property.
Lines 21-22 set the column width to 100% and the flex
value to auto
so that the columns stack on each other on small screens (1024px
breakpoint in this case).
Step 3 - Running the Website (Using CSS Flexbox)
Once done, open the index.html
file to see our nicely designed 2-column layout.

If you view the site on a smaller screen like a tablet or laptop, the page becomes responsive and automatically adjusts the columns in HTML so that they stack vertically on top of each other.

Creating a CSS Multi-Column Layout
At this point, you may wonder how to create a layout that contains more than two columns.
In this section, we'll see how to achieve that using the same properties; Grid and Flexible layout system.
First, let's see how to create a multiple columns design with Grid.
Multiple Columns Design with Grid
Step 1 - The HTML Markup (With Grid)
We'll use the same markup above, although we need to add another column in our HTML.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Two Column Layout HTML</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="./index.css">
</head>
<body>
<main>
<section class="container">
<div class="column">This is column 1.</div>
<div class="column">This is column 2.</div>
<div class="column">This is column 3.</div>
</section>
</main>
</body>
</html>
Step 2 - The CSS Style Rules (With Grid)
Using the above CSS code of Grid, adjust the existing CSS to reflect the following changes on the container
class.
.container {
display: grid;
grid-template-columns: 1fr 1fr;
gap: 1rem;
}
Change line number 3 to the following.
grid-template-columns: repeat(3, 1fr);
Or to the following.
grid-template-columns: 1fr 1fr 1fr;
Both values achieve the same result, and it's up to you to choose whichever method you prefer.
Once done, save the file and open the site.
Notice that your site now displays a three-column layout instead of two.

If you resize your browser to the 1024px
breakpoint, the columns should stack on each other.

Using the same properties above, you can increase or decrease the number of columns as you wish.
About the repeat()
CSS Function
The repeat()
CSS function allows you to specify how many columns or rows you want to repeat in your grid container.
Refer to this MDN page to read about it in detail.
Multiple Columns Design with Flexbox
Because we're using the flex
property, we don't need to make any changes to our CSS. Instead, Flexbox will automatically adjust our newly added columns in the container.
Add another column by copying and pasting the HTML for the column, as shown below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Two Column Layout HTML</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="./index.css">
</head>
<body>
<main>
<section class="container">
<div class="column">This is column 1.</div>
<div class="column">This is column 2.</div>
<div class="column">This is column 3.</div>
</section>
</main>
</body>
</html>
Save the file and open the site.
You'll now find 3 columns instead of 2.

If you resize your browser to the 1024px
breakpoint, the columns should stack on each other.

Conclusion
That's all. In this tutorial, you learned how to create responsive multiple-column website designs using the modern CSS properties; Grid and Flexbox.
If you have a suggestion or question about how to create complex multiple-column layouts, feel free to let me know via the email at [email protected].
Two Column Layout HTML FAQs
What Is a Two Column Layout?
A two column layout is one where you have two sections or columns on a website. Both columns contain information or content such as images, text, or video.
They are commonly used to create side- by - side layouts for comparison or other web design purposes.
How to Make a Two Column Layout in HTML?
To make a two column layout in HTML, follow the steps mentioned above in the tutorial.
How to Create 3 Column Layout in HTML?
To create 3 column layout in HTML or multi-column layout in HTML, follow the steps mentioned in the Creating a CSS Multi-Column Layout section of the tutorial above.
Additional Resources
Here are some related articles if you're interested in reading more about the things mentioned in this tutorial.
- Responsive Web Design: What It Is And How To Use It (Source: Smashing Magazine)
- Rem in CSS: Understanding and Using rem Units (Source: SitePoint)
- Using media queries (Source: MDN)